Table of Contents
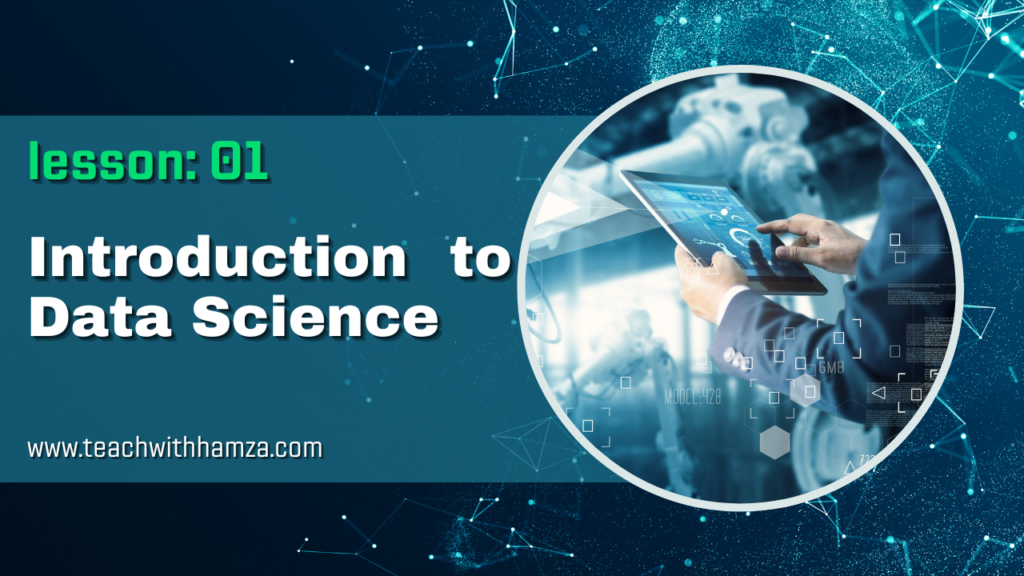
Explore our comprehensive guide on Data Science & Machine Learning. Learn about Python for data analysis, data visualization with Matplotlib and Seaborn, and dive into machine learning basics and advanced topics like deep learning and neural networks.
Chapter 1: Introduction to Data Science
Data Science has emerged as one of the most transformative fields in the modern era, revolutionizing how businesses, governments, and researchers approach data. This chapter delves into the fundamentals of Data Science, shedding light on its significance, processes, and the tools that empower professionals in this dynamic field.
1.1 What is Data Science?
Definition and Scope
Data Science is an interdisciplinary field that utilizes scientific methods, processes, algorithms, and systems to extract knowledge and insights from structured and unstructured data. It combines various techniques from statistics, machine learning, data mining, and big data technologies to analyze and interpret complex data sets.
The scope of Data Science is vast, encompassing various applications from predicting customer behavior to optimizing supply chains and advancing medical research. Its methodologies are pivotal in uncovering trends, making data-driven decisions, and deriving actionable insights.
Importance of Data Science in Today’s World
In an era where data is being generated at an unprecedented rate, Data Science plays a crucial role in converting this raw data into valuable insights. It drives innovation, enhances operational efficiency, and provides a competitive edge across multiple industries. For businesses, Data Science facilitates personalized marketing, improves customer experiences, and fosters better decision-making through predictive analytics. In healthcare, it supports disease prediction, drug discovery, and patient care optimization. Overall, the ability to leverage data effectively is a game-changer in the contemporary landscape.
Key Components: Data Collection, Data Processing, Data Analysis, and Decision-Making
- Data Collection: The first step in Data Science involves gathering raw data from various sources, such as databases, web scraping, surveys, and sensor data. Effective data collection ensures that the data is accurate, relevant, and comprehensive.
- Data Processing: Raw data often requires cleaning and transformation to prepare it for analysis. This step involves handling missing values, correcting errors, and converting data into a usable format.
- Data Analysis: This stage involves applying statistical and machine learning techniques to analyze the processed data. It includes exploratory data analysis (EDA) to understand patterns and relationships, as well as more advanced techniques to build predictive models.
- Decision-Making: The ultimate goal of Data Science is to inform decision-making. The insights derived from data analysis help stakeholders make informed choices, optimize strategies, and solve complex problems.
1.2 The Data Science Process
Problem Definition
Every Data Science project begins with a clear understanding of the problem at hand. Defining the problem involves identifying the objectives, determining the questions to be answered, and establishing success criteria. A well-defined problem ensures that the data science efforts are focused and aligned with business goals.
Data Collection
Data collection involves gathering relevant data from various sources. This can include internal databases, public datasets, and real-time data streams. The quality and quantity of data collected are crucial for the accuracy and reliability of the analysis.
Data Cleaning and Preprocessing
Raw data is often messy and incomplete. Data cleaning involves handling missing values, removing duplicates, and correcting inconsistencies. Preprocessing transforms the data into a format suitable for analysis, which may involve normalization, encoding categorical variables, and feature engineering.
Exploratory Data Analysis (EDA)
EDA is the process of analyzing data sets to summarize their main characteristics, often using visual methods. It helps in understanding the data’s structure, identifying patterns, and uncovering relationships between variables. EDA is a crucial step for formulating hypotheses and guiding further analysis.
Modeling
Modeling involves applying statistical or machine learning algorithms to the processed data to make predictions or classifications. This step includes selecting appropriate models, training them on data, and tuning hyperparameters to optimize performance.
Evaluation and Interpretation
Once models are built, they need to be evaluated to assess their effectiveness. This involves using metrics such as accuracy, precision, recall, and F1-score to measure performance. The final step is interpreting the results and translating them into actionable insights that align with the initial problem definition.
1.3 Tools and Technologies
Overview of Popular Tools: Python, R, SQL, Excel
- Python: A versatile programming language widely used in Data Science for its rich ecosystem of libraries like Pandas, NumPy, and Scikit-Learn.
- R: A statistical computing language favored for its data analysis capabilities and visualization tools.
- SQL: Essential for querying and managing relational databases.
- Excel: A spreadsheet tool used for data manipulation, basic analysis, and visualization.
Introduction to Data Science Platforms: Jupyter Notebooks, Google Colab
- Jupyter Notebooks: An open-source web application that allows for interactive computing and data visualization. It supports multiple languages, including Python, and is widely used for creating and sharing documents containing live code, equations, and visualizations.
- Google Colab: A cloud-based platform that provides free access to GPUs and enables collaborative coding with Jupyter notebooks. It’s particularly useful for developing and running machine learning models.
Cloud Services: AWS, Google Cloud, Azure
- AWS: Amazon Web Services offers a comprehensive suite of cloud-based tools and services for data storage, processing, and machine learning.
- Google Cloud: Provides various data science tools and machine learning services, including BigQuery and TensorFlow.
- Azure: Microsoft Azure offers cloud-based data storage, analytics, and machine learning services, with integrations for tools like Python and R.
1.4 Key Concepts in Data Science
Descriptive vs. Inferential Statistics
- Descriptive Statistics: Involves summarizing and describing the features of a dataset through measures like mean, median, mode, and standard deviation.
- Inferential Statistics: Involves making inferences or predictions about a population based on a sample of data. It includes hypothesis testing and confidence intervals.
Data Types: Structured, Unstructured, and Semi-Structured
- Structured Data: Organized in a fixed format, such as databases and spreadsheets.
- Unstructured Data: Lacks a predefined format, including text documents, images, and social media posts.
- Semi-Structured Data: Contains elements of both structured and unstructured data, such as JSON and XML files.
Big Data and Data Warehousing
- Big Data: Refers to large and complex data sets that cannot be easily managed or analyzed with traditional tools. It involves technologies like Hadoop and Spark for processing and analyzing massive volumes of data.
- Data Warehousing: The process of collecting, storing, and managing large amounts of data from various sources in a centralized repository. It supports data analysis and reporting.

Chapter 2: Python for Data Analysis
In the world of data science and machine learning, Python stands out as a versatile and powerful tool for data analysis. This chapter delves into the essentials of Python, its libraries, and how to leverage them for data manipulation and exploration.
2.1 Introduction to Python
Why Python for Data Analysis?
Python has become the go-to language for data analysis due to its simplicity, readability, and extensive library ecosystem. Its syntax is easy to learn, making it accessible for both beginners and experienced programmers. Additionally, Python’s libraries for data analysis and machine learning, such as Pandas, Numpy, and Scipy, are highly optimized and widely supported, enhancing its effectiveness for handling complex data tasks.
Setting Up Python Environment (Anaconda, Jupyter Notebook)
To get started with Python for data analysis, setting up a robust development environment is crucial. Anaconda is a popular distribution that simplifies package management and deployment. It comes with essential libraries and tools pre-installed. Jupyter Notebook, included in the Anaconda distribution, provides an interactive environment where you can write and execute Python code in a web-based interface. This setup is ideal for exploratory analysis and iterative coding.
Basic Python Programming Concepts: Variables, Data Types, and Operators
Before diving into data analysis, it’s important to grasp basic Python programming concepts:
- Variables: Containers for storing data values. For example,
x = 5
assigns the value5
to the variablex
. - Data Types: Python supports various data types, including integers, floats, strings, and booleans. Understanding these helps in managing and processing different types of data.
- Operators: Operators perform operations on variables and values. Python includes arithmetic operators (
+
,-
,*
,/
), comparison operators (==
,!=
,<
,>
), and logical operators (and
,or
,not
).
2.2 Python Libraries for Data Analysis
Introduction to Pandas: DataFrames, Series, and Data Manipulation
Pandas is a fundamental library for data analysis in Python. It introduces two primary data structures:
- DataFrames: 2D labeled data structures with columns of potentially different types. They are similar to tables in a database or Excel spreadsheets.
- Series: 1D labeled arrays that can hold any data type.
Pandas provides a range of functionalities for manipulating data, including reading from various file formats (CSV, Excel), filtering, grouping, merging, and aggregating data.
Numpy for Numerical Operations
Numpy, short for Numerical Python, is a library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. It is highly efficient for numerical computations and serves as the foundation for many other libraries, including Pandas and Scipy.
Scipy for Scientific Computing
Scipy builds on Numpy and offers additional functionality for scientific computing. It includes modules for optimization, integration, interpolation, eigenvalue problems, and more. Scipy is especially useful for more advanced mathematical operations that go beyond basic numerical computations.
2.3 Data Manipulation with Pandas
Reading and Writing Data (CSV, Excel, SQL)
Pandas makes it easy to import and export data in various formats:
- CSV Files: Use
pd.read_csv()
to read anddf.to_csv()
to write. - Excel Files: Use
pd.read_excel()
for reading anddf.to_excel()
for writing. - SQL Databases: Use
pd.read_sql()
to read from SQL databases anddf.to_sql()
to write data back to a database.
Data Cleaning: Handling Missing Values, Duplicates, and Data Types
Data cleaning is a critical step in data analysis. Pandas provides functions to handle missing values (df.fillna()
, df.dropna()
), remove duplicates (df.drop_duplicates()
), and convert data types (df.astype()
). Proper cleaning ensures that the data is accurate and ready for analysis.
Data Transformation: Filtering, Grouping, Merging, and Reshaping
Transforming data involves:
- Filtering: Extracting specific rows based on conditions (
df[df['column'] > value]
). - Grouping: Aggregating data based on categorical values (
df.groupby('column').mean()
). - Merging: Combining datasets using common columns or indices (
pd.merge(df1, df2, on='key')
). - Reshaping: Changing the structure of the data, such as pivoting (
df.pivot_table()
).
2.4 Exploratory Data Analysis (EDA) with Python
Descriptive Statistics
Descriptive statistics summarize the main features of a dataset. Pandas provides methods to compute statistics such as mean, median, standard deviation, and percentiles. Using df.describe()
, you can quickly get a summary of your data.
Data Visualization Basics
Data visualization is a key part of exploratory data analysis. Python libraries such as Matplotlib and Seaborn are powerful tools for creating visual representations of data.
- Matplotlib: A versatile library for creating static, animated, and interactive plots. It provides control over plot elements, such as labels and legends.
- Seaborn: Built on top of Matplotlib, Seaborn simplifies the creation of attractive and informative statistical graphics.

Chapter 3: Data Visualization (Matplotlib, Seaborn)
3.1 Introduction to Data Visualization
Importance and Benefits of Data Visualization
In the realm of Data Science and Machine Learning, the ability to visualize data effectively is paramount. Data visualization transforms raw data into intuitive graphics, making complex datasets easier to understand and analyze. By presenting data in a visual format, we can identify patterns, trends, and anomalies that might not be apparent through raw data alone.
The benefits of data visualization are manifold:
- Enhanced Clarity: Visual representations simplify complex data, enabling quicker and more accurate insights.
- Improved Communication: Effective visuals help convey findings clearly to both technical and non-technical audiences.
- Informed Decision-Making: Visualizations facilitate better decision-making by highlighting key metrics and trends.
Types of Visualizations: Charts, Plots, Graphs
Data visualization encompasses a range of formats, each serving specific purposes:
- Charts: Bar charts, pie charts, and line charts are common tools for showing comparisons and trends over time.
- Plots: Scatter plots and histograms are useful for exploring relationships between variables and distributions.
- Graphs: Network graphs and hierarchical graphs illustrate connections and structures within data.
3.2 Matplotlib
Basic Plots: Line, Bar, Scatter, Histogram
Matplotlib is a powerful Python library that provides comprehensive tools for creating static, animated, and interactive visualizations. It’s highly versatile and widely used in Data Science.
- Line Plots: Ideal for visualizing trends over time. With Matplotlib, you can easily create line plots using the
plot()
function. - Bar Charts: Useful for comparing categorical data. The
bar()
function in Matplotlib allows for the creation of vertical or horizontal bar charts. - Scatter Plots: Perfect for showing relationships between two variables. Use the
scatter()
function to plot data points and explore correlations. - Histograms: Best for displaying the distribution of a single variable. The
hist()
function provides a visual representation of data frequency.
Customizing Plots: Labels, Titles, Legends
Customizing your plots can significantly enhance their readability and interpretability:
- Labels: Use the
xlabel()
andylabel()
functions to add axis labels, providing context to your data. - Titles: Add a title to your plot with the
title()
function to summarize the visualization’s purpose. - Legends: Incorporate legends using the
legend()
function to differentiate between multiple data series in a single plot.
Advanced Plots: Subplots, 3D Plots
For more complex visualizations, Matplotlib offers advanced features:
- Subplots: Create multiple plots within a single figure using the
subplot()
function. This is useful for comparing different datasets side by side. - 3D Plots: Utilize Matplotlib’s
mplot3d
toolkit to create three-dimensional plots, ideal for visualizing complex data structures.
3.3 Seaborn
Overview of Seaborn
Seaborn is another Python library built on top of Matplotlib, designed to simplify the creation of attractive and informative statistical graphics. It integrates closely with Pandas data structures, making it an excellent choice for data scientists.
Creating Attractive Statistical Plots: Distribution Plots, Regression Plots, Heatmaps
Seaborn excels at generating sophisticated visualizations with minimal code:
- Distribution Plots: Use the
distplot()
function to visualize the distribution of a dataset, including histograms and KDE plots. - Regression Plots: Employ the
regplot()
function to display data along with a fitted regression line, helping to analyze relationships between variables. - Heatmaps: Create heatmaps using the
heatmap()
function to visualize matrix-like data, such as correlation matrices, with color-coding for intensity.
Customizing Visualizations: Themes, Color Palettes
Seaborn offers extensive customization options:
- Themes: Apply different themes using the
set_theme()
function to adjust the overall look and feel of your plots. - Color Palettes: Utilize Seaborn’s built-in color palettes or create custom palettes with the
color_palette()
function to enhance visual appeal and clarity.
3.4 Integrating Visualization with Data Analysis
Combining Matplotlib and Seaborn
Leveraging both Matplotlib and Seaborn can yield even more powerful visualizations. While Matplotlib provides extensive customization capabilities, Seaborn simplifies the creation of aesthetically pleasing statistical plots. Combining these libraries allows you to produce highly informative and visually appealing graphics.
Best Practices for Effective Visualization
To maximize the impact of your visualizations, consider these best practices:
- Clarity: Ensure your visuals are easy to interpret and free of unnecessary clutter.
- Consistency: Use consistent color schemes and formats to maintain coherence across multiple plots.
- Context: Provide sufficient context through labels, titles, and annotations to aid understanding.
In the fields of Data Science and Machine Learning, effective data visualization is crucial for deriving insights and communicating findings. By mastering tools like Matplotlib and Seaborn, you can enhance your ability to analyze and present data effectively.
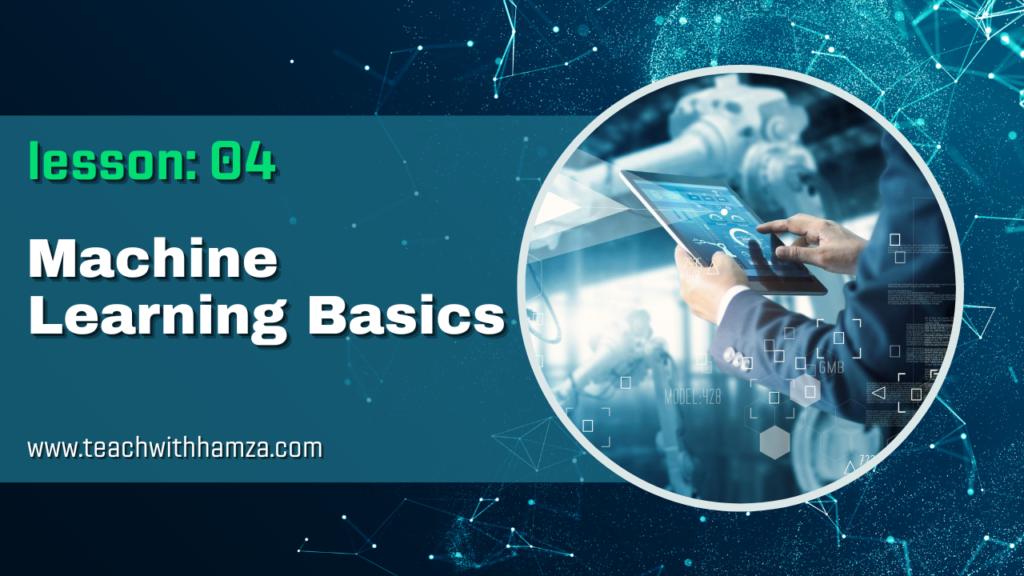
Chapter 4: Machine Learning Basics
Machine learning (ML) is a transformative technology that is reshaping how we interact with the world. From predictive analytics to intelligent assistants, machine learning is at the heart of many innovations today. In this chapter, we’ll explore the basics of machine learning, including its types, lifecycle, and key concepts such as supervised and unsupervised learning, and model evaluation. Whether you’re a newcomer or looking to solidify your understanding, this guide will provide a comprehensive overview to get you started.
4.1 Introduction to Machine Learning
Definition and Types of Machine Learning
Machine learning is a subset of artificial intelligence (AI) that enables systems to learn from data and improve their performance over time without being explicitly programmed. It is broadly categorized into three types:
- Supervised Learning: This type of learning involves training a model on a labeled dataset, where the input data and the corresponding output are both known. The goal is to learn a mapping from inputs to outputs to make predictions on new, unseen data.
- Unsupervised Learning: In unsupervised learning, the model is trained on unlabeled data. The aim is to uncover hidden patterns or structures in the data, such as grouping similar data points together or reducing data dimensionality.
- Reinforcement Learning: This type of learning involves training an agent to make a sequence of decisions by rewarding desired behaviors and penalizing undesirable ones. It is often used in scenarios where the model must learn to navigate complex environments or make decisions over time.
The Machine Learning Lifecycle
The machine learning lifecycle consists of several stages that are crucial for building effective models:
- Data Preparation: Collect and preprocess data to ensure it is clean, relevant, and suitable for analysis. This step includes handling missing values, normalizing data, and splitting it into training and test sets.
- Model Building: Select and train a machine learning model using the prepared data. This involves choosing an appropriate algorithm, configuring its parameters, and fitting it to the training data.
- Evaluation: Assess the model’s performance using metrics and validation techniques to ensure it generalizes well to new data. This step is critical for understanding how well the model will perform in real-world scenarios.
4.2 Supervised Learning
Classification vs. Regression
In supervised learning, models are typically categorized into classification or regression tasks:
- Classification: The goal is to assign inputs into predefined categories. For example, classifying emails as spam or not spam. Common classification algorithms include Logistic Regression and Support Vector Machines (SVM).
- Regression: This involves predicting a continuous output variable based on input features. For instance, forecasting house prices based on various factors like location and size. Linear Regression is a popular algorithm used for regression tasks.
Popular Algorithms
- Linear Regression: A fundamental regression algorithm that models the relationship between a dependent variable and one or more independent variables using a linear equation.
- Logistic Regression: Despite its name, it is used for classification tasks. It predicts the probability of a binary outcome based on one or more predictor variables.
- Decision Trees: A versatile algorithm that splits the data into subsets based on feature values, resulting in a tree-like structure for decision-making.
- K-Nearest Neighbors (KNN): A classification algorithm that assigns a class to an instance based on the majority class among its k-nearest neighbors in the feature space.
- Support Vector Machines (SVM): A powerful classification algorithm that finds the hyperplane that best separates different classes in the feature space.
Model Evaluation Metrics
Evaluating model performance is crucial for understanding its effectiveness. Key metrics include:
- Accuracy: The proportion of correctly classified instances out of the total instances.
- Precision: The ratio of true positive instances to the sum of true positives and false positives. It measures the quality of positive predictions.
- Recall: The ratio of true positive instances to the sum of true positives and false negatives. It indicates the model’s ability to capture all relevant instances.
- F1-Score: The harmonic mean of precision and recall, providing a single metric that balances both aspects.
4.3 Unsupervised Learning
Clustering vs. Dimensionality Reduction
Unsupervised learning focuses on discovering patterns in data without predefined labels. It is mainly categorized into:
- Clustering: Grouping data points into clusters where points in the same cluster are more similar to each other than to those in other clusters. Techniques include K-Means Clustering and Hierarchical Clustering.
- Dimensionality Reduction: Reducing the number of features in a dataset while preserving as much information as possible. Principal Component Analysis (PCA) is a common technique used for dimensionality reduction.
Popular Algorithms
- K-Means Clustering: A method that partitions data into k clusters by minimizing the variance within each cluster.
- Hierarchical Clustering: Builds a hierarchy of clusters either through agglomerative (bottom-up) or divisive (top-down) approaches.
- Principal Component Analysis (PCA): A technique that transforms data into a lower-dimensional space by finding the principal components that capture the most variance in the data.
4.4 Model Evaluation and Tuning
Cross-Validation
Cross-validation is a technique for assessing how a model generalizes to unseen data. The most common method is k-fold cross-validation, where the data is split into k subsets, and the model is trained and tested k times, each time using a different subset as the test set and the remaining as the training set.
Hyperparameter Tuning
Hyperparameters are external configurations of the learning algorithm that can significantly impact performance. Tuning involves finding the optimal values for these parameters through methods such as Grid Search or Random Search.
Overfitting and Underfitting
- Overfitting: Occurs when a model learns the training data too well, capturing noise and fluctuations rather than general patterns. This leads to poor performance on new, unseen data.
- Underfitting: Happens when a model is too simple to capture the underlying patterns in the data, resulting in poor performance on both training and test data.
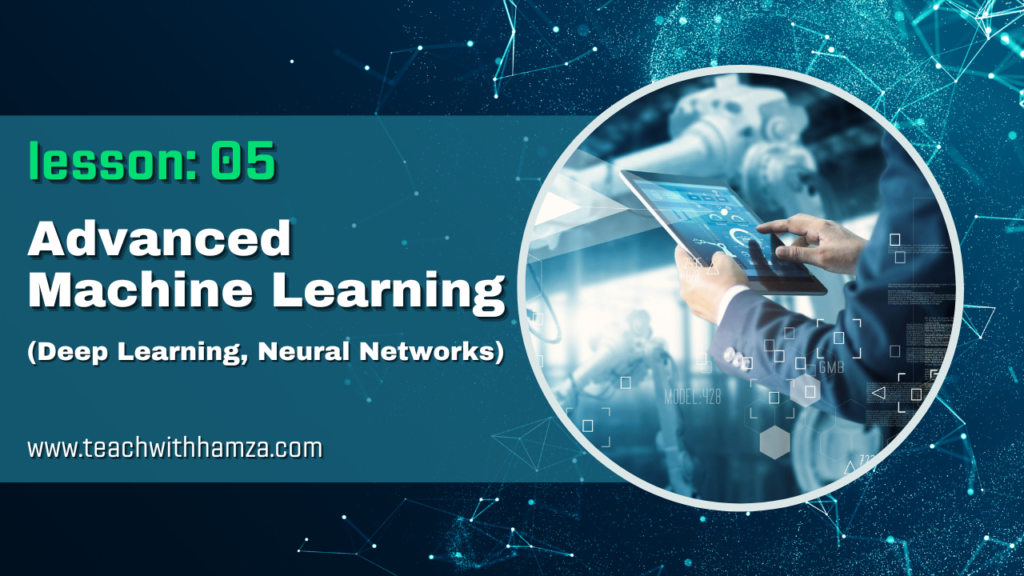
Chapter 5: Advanced Machine Learning (Deep Learning, Neural Networks)
Deep learning has revolutionized the field of machine learning, enabling the development of systems capable of understanding complex patterns and making sophisticated decisions. In this chapter, we delve into advanced topics in deep learning and neural networks, exploring their architecture, training methodologies, and applications. Whether you are an aspiring data scientist or a seasoned professional, understanding these concepts is crucial for leveraging the full potential of modern AI technologies.
5.1 Introduction to Deep Learning
Difference Between Machine Learning and Deep Learning
Machine learning (ML) encompasses a range of techniques that allow systems to learn from data and improve their performance over time. Deep learning (DL), a subset of ML, focuses on using neural networks with many layers—often referred to as deep neural networks—to model complex patterns in data.
The primary distinction between machine learning and deep learning lies in the complexity and capability of the models. While traditional ML algorithms, such as decision trees and support vector machines, require manual feature engineering and domain knowledge, deep learning models automatically learn hierarchical features from raw data. This capability enables them to excel in tasks involving unstructured data, such as images, text, and audio.
Overview of Neural Networks: Artificial Neurons, Activation Functions
Neural networks are inspired by the human brain’s structure and function. An artificial neural network consists of layers of interconnected nodes or neurons. Each neuron receives inputs, processes them, and passes the output to the next layer.
- Artificial Neurons: The fundamental building blocks of neural networks, mimicking biological neurons. Each neuron applies a linear transformation followed by a non-linear activation function to its inputs.
- Activation Functions: These functions introduce non-linearity into the network, enabling it to learn complex patterns. Common activation functions include the sigmoid, hyperbolic tangent (tanh), and Rectified Linear Unit (ReLU).
5.2 Building Neural Networks
Architecture of Neural Networks: Input Layer, Hidden Layers, Output Layer
A neural network is composed of three main types of layers:
- Input Layer: The first layer of the network, which receives the raw data. Each node in this layer represents a feature of the input data.
- Hidden Layers: Intermediate layers that perform computations and extract features from the input data. The complexity of the network increases with the number of hidden layers and neurons.
- Output Layer: The final layer that produces the network’s predictions or classifications. The output layer’s design depends on the specific task, such as regression or classification.
Common Types: Feedforward Neural Networks, Convolutional Neural Networks (CNNs), Recurrent Neural Networks (RNNs)
- Feedforward Neural Networks (FNNs): The simplest type of neural network where connections between nodes do not form cycles. FNNs are used for various tasks, including regression and classification.
- Convolutional Neural Networks (CNNs): Specialized for processing grid-like data, such as images. CNNs use convolutional layers to automatically learn spatial hierarchies of features, making them highly effective for image recognition tasks.
- Recurrent Neural Networks (RNNs): Designed for sequential data, such as time series or natural language. RNNs have connections that form cycles, allowing them to maintain context and handle variable-length sequences. Variants like Long Short-Term Memory (LSTM) and Gated Recurrent Units (GRUs) address issues like vanishing gradients in standard RNNs.
5.3 Training Deep Learning Models
Backpropagation and Optimization Algorithms (SGD, Adam)
Training deep learning models involves adjusting the network’s weights to minimize the error between predicted and actual values. This process is achieved through backpropagation and optimization algorithms.
- Backpropagation: A method used to compute gradients of the loss function with respect to each weight in the network. These gradients are then used to update the weights.
- Optimization Algorithms: Techniques like Stochastic Gradient Descent (SGD) and Adam are used to optimize the weights. SGD updates weights based on a subset of training data, while Adam adapts the learning rate based on past gradients, improving convergence.
Loss Functions and Metrics
Loss functions measure the discrepancy between predicted and actual values. Common loss functions include Mean Squared Error (MSE) for regression tasks and Cross-Entropy Loss for classification tasks. Metrics such as accuracy, precision, recall, and F1-score evaluate model performance.
5.4 Advanced Topics in Deep Learning
Transfer Learning and Pre-trained Models
Transfer learning leverages pre-trained models—networks trained on large datasets for specific tasks— as a starting point for new tasks. This approach reduces training time and improves performance by transferring learned features to new, related problems.
Generative Adversarial Networks (GANs)
GANs consist of two networks: a generator and a discriminator. The generator creates synthetic data samples, while the discriminator evaluates their authenticity. Through adversarial training, GANs generate high-quality synthetic data, which is useful in various applications, including image generation and data augmentation.
Reinforcement Learning
Reinforcement Learning (RL) focuses on training agents to make decisions by interacting with an environment. The agent learns to maximize cumulative rewards by exploring different actions and receiving feedback. RL is used in applications such as game playing, robotics, and autonomous systems.
5.5 Tools and Frameworks for Deep Learning
Introduction to TensorFlow and Keras
- TensorFlow: An open-source library developed by Google for numerical computation and deep learning. It provides comprehensive tools, libraries, and community resources for building and deploying ML models.
- Keras: A high-level API integrated with TensorFlow that simplifies the process of designing and training deep learning models. Keras offers user-friendly and modular components for rapid experimentation.
Using PyTorch for Deep Learning
PyTorch is another popular open-source deep learning framework developed by Facebook. It emphasizes dynamic computation graphs and provides a more intuitive interface for research and development. PyTorch’s flexibility and ease of use make it a favorite among researchers and practitioners.
5.6 Applications of Deep Learning
Computer Vision
Deep learning techniques, particularly CNNs, have transformed computer vision by enabling advanced image recognition, object detection, and segmentation. Applications include facial recognition, medical imaging analysis, and autonomous vehicles.
Natural Language Processing
Deep learning has significantly improved natural language processing (NLP), enabling sophisticated language models for tasks such as machine translation, sentiment analysis, and text generation. Technologies like transformers and attention mechanisms have been pivotal in this progress.
Speech Recognition
Deep learning models have revolutionized speech recognition by achieving high accuracy in transcribing spoken language. Applications range from voice assistants and transcription services to real-time language translation.